Note
Go to the end to download the full example code.
Distribution Class
Handles the initialization of different statistical distribution
from geobipy import Distribution
from geobipy import plotting as cP
import matplotlib.pyplot as plt
import numpy as np
from numpy.random import Generator
from numpy.random import PCG64DXSM
generator = PCG64DXSM(seed=0)
prng = Generator(generator)
Univariate Normal Distribution
D = Distribution('Normal', 0.0, 1.0, prng=prng)
# Get the bins of the Distribution from +- 4 standard deviations of the mean
bins = D.bins()
# Grab random samples from the distribution
D.rng(10)
# We can then get the Probability Density Function for those bins
pdf = D.probability(bins, log=False)
# And we can plot that PDF
plt.figure()
plt.plot(bins, pdf)
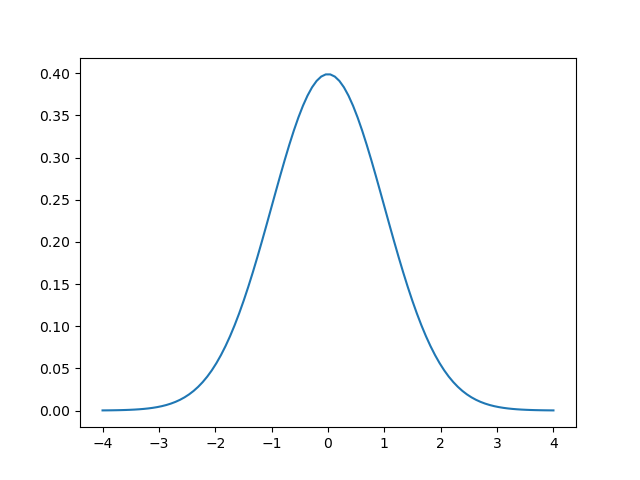
[<matplotlib.lines.Line2D object at 0x17f679190>]
Multivariate Normal Distribution
D = Distribution('MvNormal',[0.0,1.0,2.0],[1.0,1.0,1.0], prng=prng)
D.rng()
array([ 0.64050649, 1.77177243, -0.34500474])
Uniform Distribution
D = Distribution('Uniform', 0.0, 1.0, prng=prng)
D.bins()
DataArray([0. , 0.01010101, 0.02020202, ..., 0.97979798,
0.98989899, 1. ])
Total running time of the script: (0 minutes 0.050 seconds)