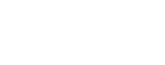
Plot monthly frequency analysis
monthly_frequency_plot.Rd
This plot uses calculations from monthly_frequency_table
. Daily, discrete,
or both types of data can be included.
Usage
monthly_frequency_plot(
gw_level_dv,
gwl_data,
parameter_cd = NA,
date_col = NA,
value_col = NA,
approved_col = NA,
stat_cd = NA,
plot_title = "",
subtitle = "U.S. Geological Survey",
plot_range = c("Past year"),
y_axis_label = "",
flip = FALSE,
percentile_colors = NA,
include_edges = FALSE,
median_point_size = 2.5,
data_point_size = 2.5
)
Arguments
- gw_level_dv
data frame, daily groundwater level data. Often obtained from
readNWISdv
. UseNULL
for no daily data.- gwl_data
data frame returned from
readNWISgwl
, or data frame with a date, value, and approval columns. Using the convention: lev_dt (representing date), lev_age_cd (representing approval code), and lev_va or sl_lev_va (representing value) will allow defaults to work. UseNULL
for no discrete data.- parameter_cd
If data in gw_level_dv comes from NWIS, the parameter_cd can be used to define the value_col. If the data doesn't come directly from NWIS services, this can be set to
NA
,and this argument will be ignored.- date_col
the name of the date column. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the date column for gw_level_dv, and the second defines gwl_data.- value_col
the name of the value column. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the value column for gw_level_dv, and the second defines gwl_data.- approved_col
the name of the column to get provisional/approved status. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the approval column for gw_level_dv, and the second defines gwl_data.- stat_cd
If data in gw_level_dv comes from NWIS, the stat_cd can be used to help define the value_col.
- plot_title
the title to use on the plot.
- subtitle
character. Sub-title for plot, default is "U.S. Geological Survey".
- plot_range
the time frame to use for the plot. Either "Past year" to use the last year of data, or "Calendar year" to use the current calendar year, beginning in January.
- y_axis_label
the label used for the y-axis of the plot.
- flip
logical. If
TRUE
, flips the y axis so that the smallest number is on top. Default isTRUE
.- percentile_colors
Optional argument to provide a vector of 5 or 7 colors used to fill the percentile bars in order from lowest percentile bin to the highest percentile bin. Default behavior (
NA
) is to use legacy plot colors. If include_edges parameter is set to TRUE, then this vector must be 7 colors long.- include_edges
Optional argument to toggle on the "edge bins" min-5 and 95-max on the plot. Default is FALSE which does not plot those bins.
- median_point_size
Optional argument to specify the size of the median point markers which are shown as black triangles on the plot. The default size is 2.5.
- data_point_size
Optional argument to specify the size of the data point markers which are shown as red diamonds on the plot. The default size is 2.5.
Value
a ggplot with rectangles representing the historical monthly percentile, black triangles representing the historical monthly median, and red diamonds showing the last year of groundwater level measurements.
Examples
# site <- "263819081585801"
p_code_dv <- "62610"
statCd <- "00001"
# gw_level_dv <- dataRetrieval::readNWISdv(site, p_code_dv, statCd = statCd)
gw_level_dv <- L2701_example_data$Daily
label <- dataRetrieval::readNWISpCode(p_code_dv)[["parameter_nm"]]
monthly_frequency <- monthly_frequency_plot(gw_level_dv,
gwl_data = NULL,
parameter_cd = "62610",
plot_title = "L2701 Groundwater Level",
y_axis_label = label,
flip = FALSE)
monthly_frequency
gwl_data <- L2701_example_data$Discrete
monthly_frequency_plot(gw_level_dv,
gwl_data = gwl_data,
parameter_cd = "62610",
plot_title = "L2701 Groundwater Level",
y_axis_label = label,
flip = FALSE)
monthly_frequency_flip <- monthly_frequency_plot(gw_level_dv,
gwl_data,
parameter_cd = "62610",
y_axis_label = label,
plot_title = "L2701 Groundwater Level",
flip = TRUE)
monthly_frequency_flip
monthly_frequency_custom_colors <- monthly_frequency_plot(gw_level_dv,
gwl_data,
parameter_cd = "62610",
y_axis_label = label,
plot_title = "L2701 Groundwater Level",
flip = TRUE,
percentile_colors = c(
"red",
"yellow",
"green",
"blue",
"orange"
))
monthly_frequency_custom_colors
monthly_frequency_edge_bins <- monthly_frequency_plot(gw_level_dv,
gwl_data,
parameter_cd = "62610",
y_axis_label = label,
plot_title = "L2701 Groundwater Level",
flip = FALSE,
include_edges = TRUE)
monthly_frequency_edge_bins
monthly_frequency_custom_point_sizes <- monthly_frequency_plot(gw_level_dv,
gwl_data = gwl_data,
parameter_cd = "62610",
plot_title = "L2701 Groundwater Level",
y_axis_label = label,
median_point_size = 0.5,
data_point_size = 3)
monthly_frequency_custom_point_sizes