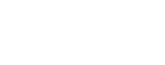
Create a table of monthly frequency analysis
monthly_frequency_table.Rd
The table will accept daily, discrete, or a both types of data. The median of each
year/month is calculated. Then using that median, monthly stats are calculated.
Percentiles are calculated using the quantile
function with "type=6".
Usage
monthly_frequency_table(
gw_level_dv,
gwl_data,
parameter_cd = NA,
date_col = NA,
value_col = NA,
approved_col = NA,
stat_cd = NA,
flip = FALSE
)
Arguments
- gw_level_dv
data frame, daily groundwater level data. Often obtained from
readNWISdv
. UseNULL
for no daily data.- gwl_data
data frame returned from
readNWISgwl
, or data frame with a date, value, and approval columns. Using the convention: lev_dt (representing date), lev_age_cd (representing approval code), and lev_va or sl_lev_va (representing value) will allow defaults to work. UseNULL
for no discrete data.- parameter_cd
If data in gw_level_dv comes from NWIS, the parameter_cd can be used to define the value_col. If the data doesn't come directly from NWIS services, this can be set to
NA
,and this argument will be ignored.- date_col
the name of the date column. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the date column for gw_level_dv, and the second defines gwl_data.- value_col
the name of the value column. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the value column for gw_level_dv, and the second defines gwl_data.- approved_col
the name of the column to get provisional/approved status. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the approval column for gw_level_dv, and the second defines gwl_data.- stat_cd
If data in gw_level_dv comes from NWIS, the stat_cd can be used to help define the value_col.
- flip
logical. If
TRUE
, flips labels so that the lower numbers are in the higher percentages. Default isTRUE
.
Value
a data frame of monthly groundwater level statistics including the 5th, 10th, 25th, 75th, 90th, and 95th percentiles; the number of years of data; and the lowest monthly median and the highest monthly median.
Examples
# site <- "263819081585801"
p_code_dv <- "62610"
statCd <- "00001"
# gw_level_dv <- dataRetrieval::readNWISdv(site, p_code_dv, statCd = statCd)
gw_level_dv <- L2701_example_data$Daily
monthly_frequency <- monthly_frequency_table(gw_level_dv,
NULL,
parameter_cd = "62610")
head(monthly_frequency)
#> # A tibble: 6 × 11
#> month p05 p10 p25 p50 p75 p90 p95 nYears minMed maxMed
#> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dbl>
#> 1 1 -38.8 -32.3 -29.5 -21.5 -17.8 -12.2 -6.88 42 -41.8 -5.68
#> 2 2 -41.2 -32.6 -29.1 -22.1 -19.0 -12.6 -7.53 43 -42.8 -7.25
#> 3 3 -41.4 -36.6 -30.0 -24.3 -20.4 -10.2 -7.65 42 -45.1 -6.87
#> 4 4 -45.6 -39.2 -32.2 -26.4 -22.4 -16.1 -10.1 42 -48.5 -9.34
#> 5 5 -46.5 -40.0 -34.3 -29.2 -23.5 -18.2 -14.2 42 -49.4 -10.0
#> 6 6 -42.0 -39.4 -32.4 -28.3 -21.3 -17.0 -13.6 41 -42.8 -11.0
gwl_data <- L2701_example_data$Discrete
monthly_frequency_combo <- monthly_frequency_table(gw_level_dv,
gwl_data,
parameter_cd = "62610")
head(monthly_frequency_combo)
#> # A tibble: 6 × 11
#> month p05 p10 p25 p50 p75 p90 p95 nYears minMed maxMed
#> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dbl>
#> 1 1 -38.6 -32.3 -29.5 -21.6 -17.9 -12.6 -6.95 43 -41.8 -5.68
#> 2 2 -41.2 -32.6 -29.1 -22.1 -19.0 -12.5 -7.53 43 -42.9 -7.25
#> 3 3 -41.5 -36.4 -30.0 -24 -19.8 -10.6 -7.68 43 -45.2 -6.87
#> 4 4 -45.7 -39.2 -32.2 -26.4 -22.6 -16.1 -10.1 42 -48.4 -9.36
#> 5 5 -46.5 -40.0 -34.3 -29.4 -23.5 -18.2 -14.2 42 -49.4 -10.0
#> 6 6 -42.0 -39.4 -32.5 -28.4 -21.7 -17.0 -13.6 41 -42.8 -11.0
monthly_flip <- monthly_frequency_table(gw_level_dv,
gwl_data,
parameter_cd = "62610",
flip = TRUE)
head(monthly_flip)
#> # A tibble: 6 × 11
#> month p05 p10 p25 p50 p75 p90 p95 nYears minMed maxMed
#> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dbl>
#> 1 1 -6.95 -12.6 -17.9 -21.6 -29.5 -32.3 -38.6 43 -5.68 -41.8
#> 2 2 -7.53 -12.5 -19.0 -22.1 -29.1 -32.6 -41.2 43 -7.25 -42.9
#> 3 3 -7.68 -10.6 -19.8 -24 -30.0 -36.4 -41.5 43 -6.87 -45.2
#> 4 4 -10.1 -16.1 -22.6 -26.4 -32.2 -39.2 -45.7 42 -9.36 -48.4
#> 5 5 -14.2 -18.2 -23.5 -29.4 -34.3 -40.0 -46.5 42 -10.0 -49.4
#> 6 6 -13.6 -17.0 -21.7 -28.4 -32.5 -39.4 -42.0 41 -11.0 -42.8