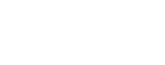
Create a table of weekly frequency analysis
weekly_frequency_table.Rd
The weekly frequency analysis is based on daily, discrete, or both types of data. The median of each year/week combo is calculated, then overall weekly statistics are calculated off of that median.
Usage
weekly_frequency_table(
gw_level_dv,
gwl_data,
parameter_cd = NA,
date_col = NA,
value_col = NA,
approved_col = NA,
stat_cd = NA,
flip = FALSE
)
Arguments
- gw_level_dv
data frame, daily groundwater level data. Often obtained from
readNWISdv
. UseNULL
for no daily data.- gwl_data
data frame returned from
readNWISgwl
, or data frame with a date, value, and approval columns. Using the convention: lev_dt (representing date), lev_age_cd (representing approval code), and lev_va or sl_lev_va (representing value) will allow defaults to work. UseNULL
for no discrete data.- parameter_cd
If data in gw_level_dv comes from NWIS, the parameter_cd can be used to define the value_col. If the data doesn't come directly from NWIS services, this can be set to
NA
,and this argument will be ignored.- date_col
the name of the date column. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the date column for gw_level_dv, and the second defines gwl_data.- value_col
the name of the value column. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the value column for gw_level_dv, and the second defines gwl_data.- approved_col
the name of the column to get provisional/approved status. The default is
NA
, in which case, the code will try to get the column name automatically based on NWIS naming conventions. If both gw_level_dv and gwl_data data frames require custom column names, the first value of this input defines the approval column for gw_level_dv, and the second defines gwl_data.- stat_cd
If data in gw_level_dv comes from NWIS, the stat_cd can be used to help define the value_col.
- flip
logical. If
TRUE
, flips labels so that the lower numbers are in the higher percentages. Default isTRUE
.
Examples
# site <- "263819081585801"
p_code_dv <- "62610"
statCd <- "00001"
# gw_level_dv <- dataRetrieval::readNWISdv(site, p_code_dv, statCd = statCd)
gw_level_dv <- L2701_example_data$Daily
weekly_frequency <- weekly_frequency_table(gw_level_dv,
NULL,
parameter_cd = "62610")
head(weekly_frequency)
#> # A tibble: 6 × 12
#> week p05 p10 p25 p50 p75 p90 p95 nYears minMed maxMed
#> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dbl>
#> 1 1 -38.5 -33.1 -28.8 -21.4 -17.5 -12.3 -8.59 41 -40.6 -5.48
#> 2 2 -39.3 -32.8 -29.3 -21.4 -17.5 -12.3 -7.38 42 -41.4 -5.52
#> 3 3 -38.8 -32.6 -29.2 -21.2 -17.7 -12.2 -6.11 42 -42.1 -5.68
#> 4 4 -39.4 -32.0 -29.7 -21.4 -18.3 -12.0 -6.83 42 -41.9 -6.12
#> 5 5 -40.4 -32.1 -29.7 -21.7 -18.6 -12.4 -7.01 43 -41.6 -6.8
#> 6 6 -41.0 -32.3 -29.0 -21.9 -19.0 -12.4 -7.89 43 -42.1 -7.02
#> # ℹ 1 more variable: week_start <chr>
gwl_data <- L2701_example_data$Discrete
weekly_frequency <- weekly_frequency_table(gw_level_dv,
gwl_data,
parameter_cd = "62610")
weekly_frequency
#> # A tibble: 53 × 12
#> week p05 p10 p25 p50 p75 p90 p95 nYears minMed maxMed
#> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dbl>
#> 1 1 -38.5 -33.1 -28.9 -21.4 -17.5 -12.3 -8.59 41 -40.6 -5.48
#> 2 2 -39.3 -32.8 -29.3 -21.4 -17.5 -12.3 -7.38 42 -41.4 -5.52
#> 3 3 -38.8 -32.6 -29.2 -21.2 -17.7 -12.2 -6.11 42 -42.1 -5.68
#> 4 4 -39.5 -32.1 -29.8 -21.5 -18.3 -12.1 -6.83 42 -42.4 -6.12
#> 5 5 -40.4 -32.1 -29.7 -21.7 -18.6 -12.4 -7.01 43 -41.6 -6.8
#> 6 6 -41.0 -32.3 -29.0 -21.9 -19.0 -12.4 -7.89 43 -42.1 -7.02
#> 7 7 -40.8 -32.6 -29.2 -22.2 -19 -12.8 -7.69 43 -43.4 -7.45
#> 8 8 -41.2 -33.6 -29.3 -22.2 -18.6 -11.2 -7.80 43 -45.0 -6.07
#> 9 9 -41.7 -34.8 -29.7 -22.4 -19.3 -10.3 -7.88 43 -42.6 -6.15
#> 10 10 -41.1 -35.3 -30.0 -23.3 -19.9 -9.29 -7.64 42 -43.3 -6.26
#> # ℹ 43 more rows
#> # ℹ 1 more variable: week_start <chr>
weekly_flip <- weekly_frequency_table(gw_level_dv,
gwl_data,
parameter_cd = "62610",
flip = TRUE)
weekly_flip
#> # A tibble: 53 × 12
#> week p05 p10 p25 p50 p75 p90 p95 nYears minMed maxMed
#> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <dbl> <int> <dbl> <dbl>
#> 1 1 -8.59 -12.3 -17.5 -21.4 -28.9 -33.1 -38.5 41 -5.48 -40.6
#> 2 2 -7.38 -12.3 -17.5 -21.4 -29.3 -32.8 -39.3 42 -5.52 -41.4
#> 3 3 -6.11 -12.2 -17.7 -21.2 -29.2 -32.6 -38.8 42 -5.68 -42.1
#> 4 4 -6.83 -12.1 -18.3 -21.5 -29.8 -32.1 -39.5 42 -6.12 -42.4
#> 5 5 -7.01 -12.4 -18.6 -21.7 -29.7 -32.1 -40.4 43 -6.8 -41.6
#> 6 6 -7.89 -12.4 -19.0 -21.9 -29.0 -32.3 -41.0 43 -7.02 -42.1
#> 7 7 -7.69 -12.8 -19 -22.2 -29.2 -32.6 -40.8 43 -7.45 -43.4
#> 8 8 -7.80 -11.2 -18.6 -22.2 -29.3 -33.6 -41.2 43 -6.07 -45.0
#> 9 9 -7.88 -10.3 -19.3 -22.4 -29.7 -34.8 -41.7 43 -6.15 -42.6
#> 10 10 -7.64 -9.29 -19.9 -23.3 -30.0 -35.3 -41.1 42 -6.26 -43.3
#> # ℹ 43 more rows
#> # ℹ 1 more variable: week_start <chr>