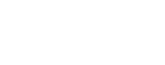
Function to return data from the NWISWeb WaterML1.1 service
Source:R/importWaterML1.R
importWaterML1.Rd
This function accepts a url parameter that already contains the desired NWIS site, parameter code, statistic, startdate and enddate.
Arguments
- obs_url
character or raw, containing the url for the retrieval or a file path to the data file, or raw XML.
- asDateTime
logical, if
TRUE
returns date and time as POSIXct, ifFALSE
, Date- tz
character to set timezone attribute of datetime. Default converts the datetimes to UTC (properly accounting for daylight savings times based on the data's provided tz_cd column). Recommended US values include "UTC", "America/New_York", "America/Chicago", "America/Denver", "America/Los_Angeles", "America/Anchorage", "America/Honolulu", "America/Jamaica", "America/Managua", "America/Phoenix", and "America/Metlakatla". For a complete list, see https://en.wikipedia.org/wiki/List_of_tz_database_time_zones
Value
A data frame with the following columns:
Name | Type | Description |
agency_cd | character | The NWIS code for the agency reporting the data |
site_no | character | The USGS site number |
POSIXct | The date and time of the value converted to UTC (if asDateTime = TRUE), | |
character | or raw character string (if asDateTime = FALSE) | |
tz_cd | character | The time zone code for |
code | character | Any codes that qualify the corresponding value |
value | numeric | The numeric value for the parameter |
Note that code and value are repeated for the parameters requested. The names are of the form X_D_P_S, where X is literal, D is an option description of the parameter, P is the parameter code, and S is the statistic code (if applicable).
There are also several useful attributes attached to the data frame:
Name | Type | Description |
url | character | The url used to generate the data |
siteInfo | data.frame | A data frame containing information on the requested sites |
variableInfo | data.frame | A data frame containing information on the requested parameters |
statisticInfo | data.frame | A data frame containing information on the requested statistics on the data |
queryTime | POSIXct | The time the data was returned |
Examples
site_id <- "02177000"
startDate <- "2012-09-01"
endDate <- "2012-10-01"
offering <- "00003"
property <- "00060"
obs_url <- constructNWISURL(site_id, property, startDate, endDate, "dv")
# \donttest{
data <- importWaterML1(obs_url, asDateTime = TRUE)
#> GET: https://waterservices.usgs.gov/nwis/dv/?site=02177000&format=waterml%2C1.1&ParameterCd=00060&StatCd=00003&startDT=2012-09-01&endDT=2012-10-01
unitDataURL <- constructNWISURL(
site_id, property,
"2013-11-03", "2013-11-03", "uv"
)
unitData <- importWaterML1(unitDataURL, TRUE)
#> GET: https://nwis.waterservices.usgs.gov/nwis/iv/?site=02177000&format=waterml%2C1.1&ParameterCd=00060&startDT=2013-11-03&endDT=2013-11-03
# Two sites, two pcodes, one site has two data descriptors:
siteNumber <- c("01480015", "04085427")
obs_url <- constructNWISURL(
siteNumber, c("00060", "00010"),
startDate, endDate, "dv"
)
data <- importWaterML1(obs_url)
#> GET: https://waterservices.usgs.gov/nwis/dv/?site=01480015%2C04085427&format=waterml%2C1.1&ParameterCd=00060%2C00010&StatCd=00003&startDT=2012-09-01&endDT=2012-10-01
data$dateTime <- as.Date(data$dateTime)
data <- renameNWISColumns(data)
names(attributes(data))
#> [1] "names" "row.names" "url" "siteInfo"
#> [5] "variableInfo" "disclaimer" "statisticInfo" "queryTime"
#> [9] "class"
attr(data, "url")
#> [1] "https://waterservices.usgs.gov/nwis/dv/?site=01480015%2C04085427&format=waterml%2C1.1&ParameterCd=00060%2C00010&StatCd=00003&startDT=2012-09-01&endDT=2012-10-01"
attr(data, "disclaimer")
#> [1] "Provisional data are subject to revision. Go to http://waterdata.usgs.gov/nwis/help/?provisional for more information."
inactiveSite <- "05212700"
inactiveSite <- constructNWISURL(inactiveSite, "00060",
"2014-01-01", "2014-01-10", "dv")
inactiveSite <- importWaterML1(inactiveSite)
#> GET: https://waterservices.usgs.gov/nwis/dv/?site=05212700&format=waterml%2C1.1&ParameterCd=00060&StatCd=00003&startDT=2014-01-01&endDT=2014-01-10
inactiveAndAcitive <- c("07334200", "05212700")
inactiveAndAcitive <- constructNWISURL(inactiveAndAcitive,
"00060", "2014-01-01", "2014-01-10", "dv")
inactiveAndAcitive <- importWaterML1(inactiveAndAcitive)
#> GET: https://waterservices.usgs.gov/nwis/dv/?site=07334200%2C05212700&format=waterml%2C1.1&ParameterCd=00060&StatCd=00003&startDT=2014-01-01&endDT=2014-01-10
# Timezone change with specified local timezone:
tzURL <- constructNWISURL("04027000", c("00300", "63680"),
"2011-11-05", "2011-11-07", "uv")
tzIssue <- importWaterML1(tzURL,
asDateTime = TRUE, tz = "America/Chicago"
)
#> GET: https://nwis.waterservices.usgs.gov/nwis/iv/?site=04027000&format=waterml%2C1.1&ParameterCd=00300%2C63680&startDT=2011-11-05&endDT=2011-11-07
# }
filePath <- system.file("extdata", package = "dataRetrieval")
fileName <- "WaterML1Example.xml"
fullPath <- file.path(filePath, fileName)
importFile <- importWaterML1(fullPath, TRUE)